Emission
Emission is the process by which the Bittensor network allocates TAO and alpha to participants, including miners, validators, stakers, and subnet creators.
It unfolds in two stages:
- Injection into subnets
- Extraction by participants
See the Dynamic TAO White Paper for a full explanation.
Injection
The first stage of emissions is injection of liquidity into the subnet pools. Liquidity is injected to each subnet in proportion to the price of its token compared to the price of other subnet tokens. This is designed to incentivize development on the most valuable subnets.
Each block:
- TAO is injected into the subnet's TAO reserve.
- Alpha is injected into the subnet's alpha reserve.
- Alpha is allocated to alpha outstanding, to be extracted by participants.
TAO reserve injection
A subnet's TAO reserve injection is computed in proportion to the price of its alpha token over the sum of prices for all the subnets in Bittensor.
Given set S of all subnets, and a total per block TAO emission , which begins at 1 TAO and follows a halving schedule, TAO emission to subnet with price is:
Alpha reserve injection
Alpha is then injected in proportion to the price of the token, so that growth of a subnet's liquidity pools does not not change the price of the alpha token.
Recall that token price for a subnet is its TAO in reserve divided by its alpha reserve:
So in order to inject alpha without changing the price, it should follow:
When we fill in this equation with the previous formula for , the price is cancelled out of the equation, yielding:
However, alpha injection is also capped at 1 by the algorithm, to prevent runaway inflation. Therefore, with cap or alpha emission rate , emission to subnet is:
The cap or alpha emission rate for subnet , starts at 1 and follows a halving schedule identical to that of TAO, beginning when subnet is created.
Alpha outstanding injection
Each block, liquidity is also set aside to be emitted to participants (validators, miners, stakers, and subnet creator). The quantity per block is equal to the alpha emission rate for subnet .
Extraction
At the end of each tempo (360 blocks), the quantity of alpha accumulated over each block of the tempo is extracted by network participants in the following proportions:
-
18% by subnet owner
-
41% of emissions go to miners. The allocation to particular miners is determined by Yuma Consensus: Miner emissions#miner-emissions.
-
41% by validators and their stakers.
-
First, the allocation to validators miners is determined by Yuma Consensus: Validator Emissions.
-
Then, validators extract their take from that allocation.
-
Then, TAO and alpha are emitted to stakers in proportion to the validators' holdings in each token. TAO emissions are sourced by swapping a portion of alpha emissions to TAO through the subnet's liquidity pool.
For validator x's TAO stake , and alpha stake , and the global TAO weight :
-
TAO is emitted to stakers on the root subnet (i.e. stakers in TAO) in proportion to the validator's stake weight's proportion of TAO.
-
Alpha is emitted to stakers on the mining subnet (i.e. stakers in alpha) in proportion to the validator's stake weight's proportion of alpha:
Validators who hold both root TAO and subnet alphas will extract both types of token.
-
-
Note on evolution of Bittensor token economy
When Dynamic TAO is initiated, there will be no alpha in circulation, so validator's stake weights will be entirely determined by their share of TAO stake.
But far more alpha than TAO is emitted into circulation every block. As a result, over time there will be more alpha relative to TAO in overall circulation, and the relative weight of a validator in a given subnet will depend more on their alpha stake share relative to their share of the TAO stake on Subnet Zero.
In order to hasten the process of alpha gaining the majority of stake power in the network, the contribution of TAO stake to validator stake weight is reduced by a global parameter called TAO weight. Currently, this is planned to be 18%, in order to achieve a weight parity between TAO and total alpha in approximately 100 days.
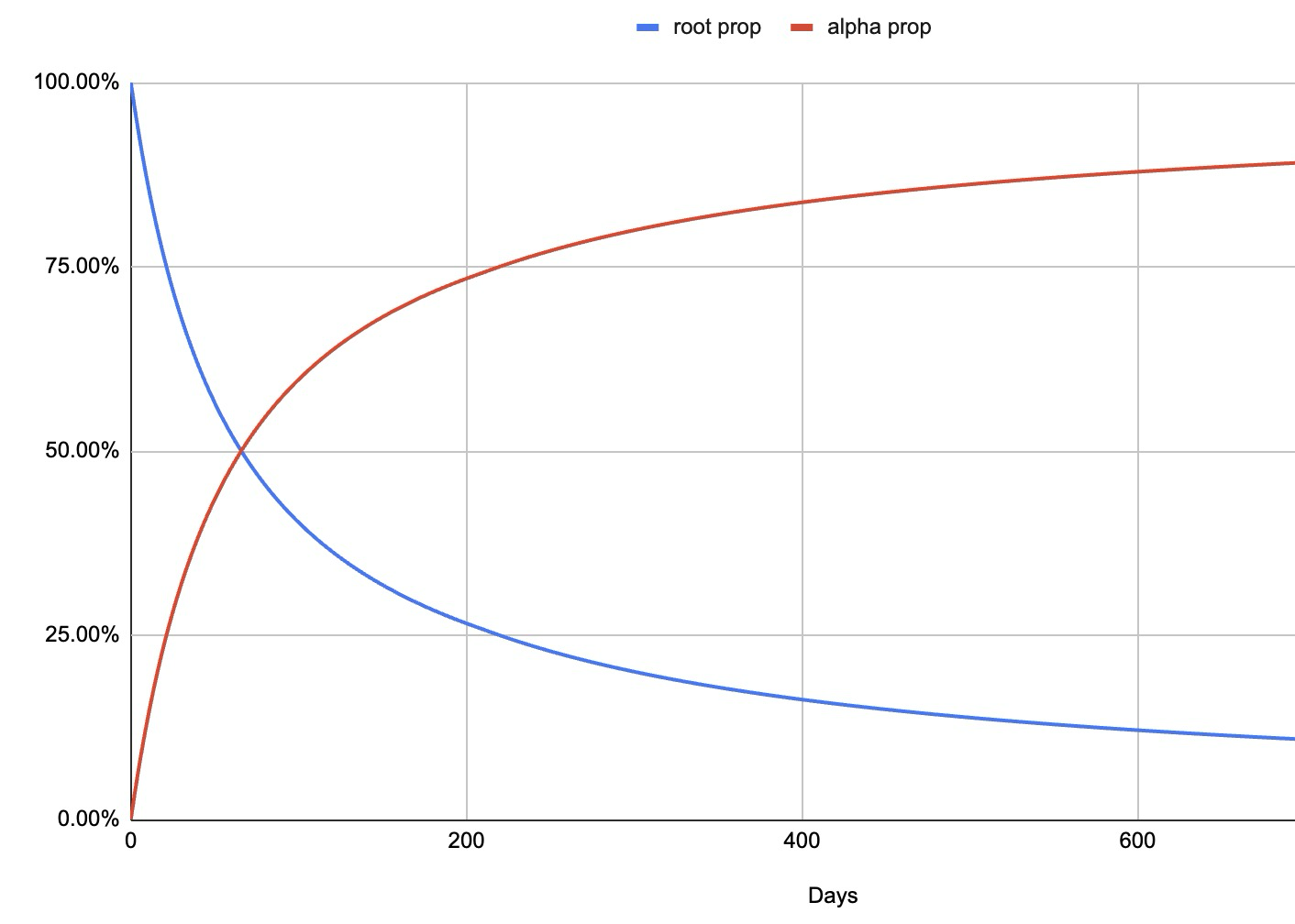
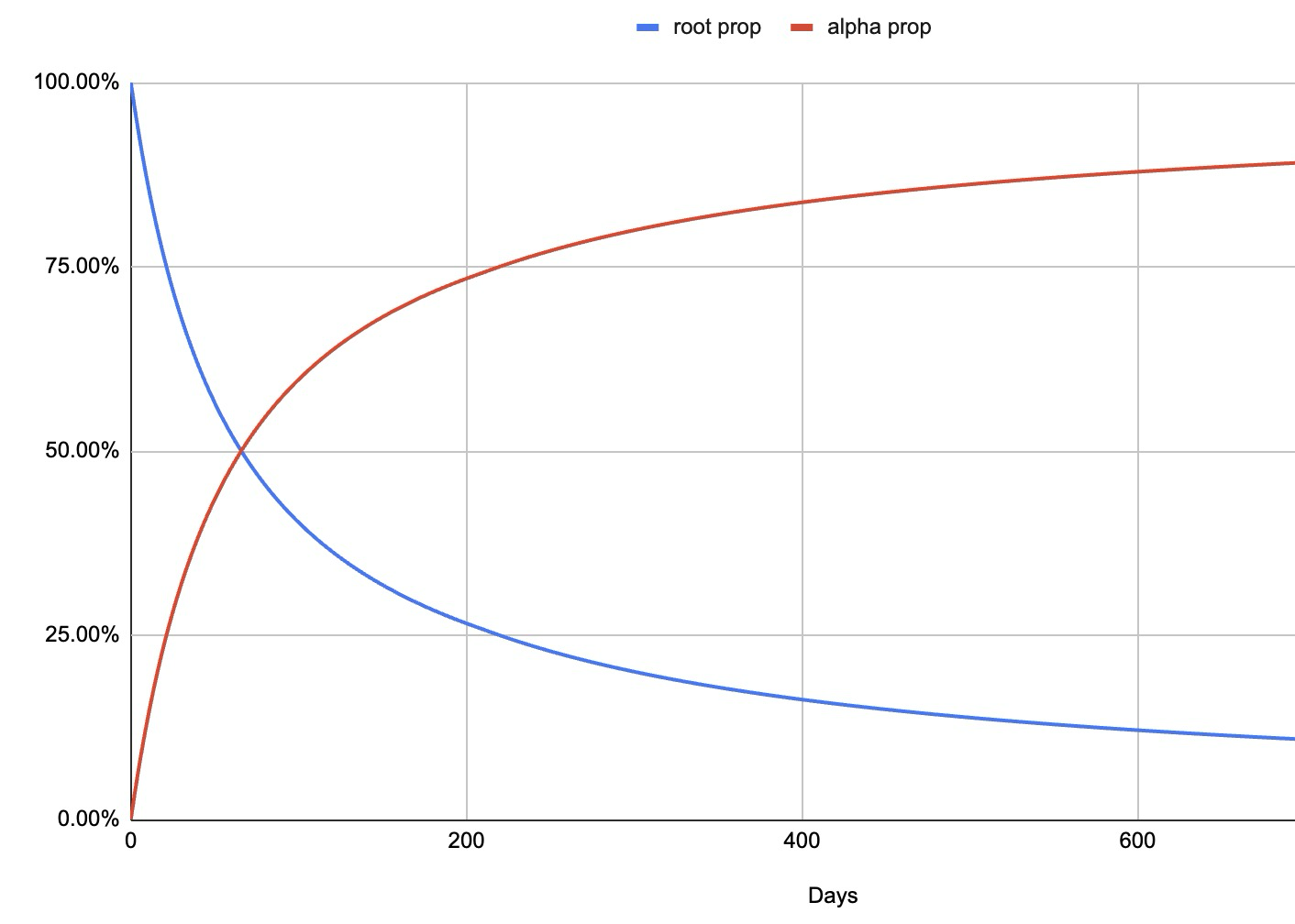